티스토리 뷰
728x90
반응형
[1] Device Info
Flutter에서 공식 제공하는 device_info 패키지를 사용하면 됩니다.
패키지 설치 후, 예제코드 를 참고해서 구현해주겠습니다.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import 'package:device_info/device_info.dart'; | |
Future<Map<String, dynamic>> _getDeviceInfo() async { | |
DeviceInfoPlugin deviceInfoPlugin = DeviceInfoPlugin(); | |
Map<String, dynamic> deviceData = <String, dynamic>{}; | |
try { | |
if (Platform.isAndroid) { | |
deviceData = _readAndroidDeviceInfo(await deviceInfoPlugin.androidInfo); | |
} else if (Platform.isIOS) { | |
deviceData = _readIosDeviceInfo(await deviceInfoPlugin.iosInfo); | |
} | |
} catch(error) { | |
deviceData = { | |
"Error": "Failed to get platform version." | |
}; | |
} | |
return deviceData; | |
} | |
Map<String, dynamic> _readAndroidDeviceInfo(AndroidDeviceInfo info) { | |
var release = info.version.release; | |
var sdkInt = info.version.sdkInt; | |
var manufacturer = info.manufacturer; | |
var model = info.model; | |
return { | |
"OS 버전": "Android $release (SDK $sdkInt)", | |
"기기": "$manufacturer $model" | |
}; | |
} | |
Map<String, dynamic> _readIosDeviceInfo(IosDeviceInfo info) { | |
var systemName = info.systemName; | |
var version = info.systemVersion; | |
var machine = info.utsname.machine; | |
return { | |
"OS 버전": "$systemName $version", | |
"기기": "$machine" | |
}; | |
} | |
// 실행 코드 | |
Map<String, dynamic> deviceInfo = await _getDeviceInfo(); | |
print(deviceInfo) | |
// 실행 결과 | |
// 안드로이드 | |
{ | |
"OS 버전": "Android 11 (SDK 30)" | |
"기기": "Google sdk_gphone_x86_arm" | |
} | |
// iOS | |
{ | |
"OS 버전": "iOS 14.4" | |
"기기": "iPhone12,2" | |
} | |
iOS 기기 정보가 예상한 값이 아니죠...?
www.theiphonewiki.com/wiki/Models 여기보면
Generation과 Identifier가 각각 있는데요,
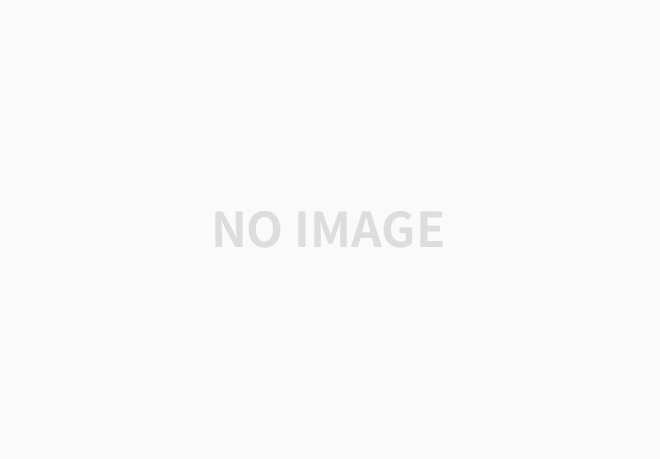
위 패키지에서는
Generation을 알려주는 필드는 없고 Identifier를 알려주는 필드만 있습니다 🥲🥲
위의 실행결과에서 iPhone12,1 을 보고
iPhone11이구나! 하고 알아야하는 것입니다..!
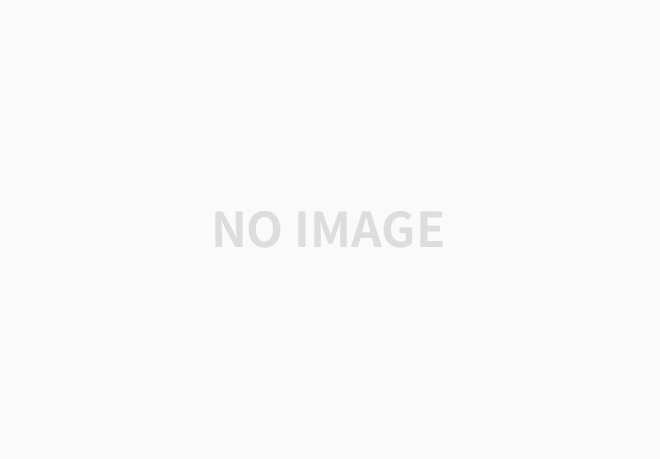
하지만 이렇게 매칭하기 힘드니까
pub.dev/packages/ios_utsname_ext 패키지를 쓸 수 있습니다.
이 패키지는 iOSProductionName이라는 extension을 제공합니다.
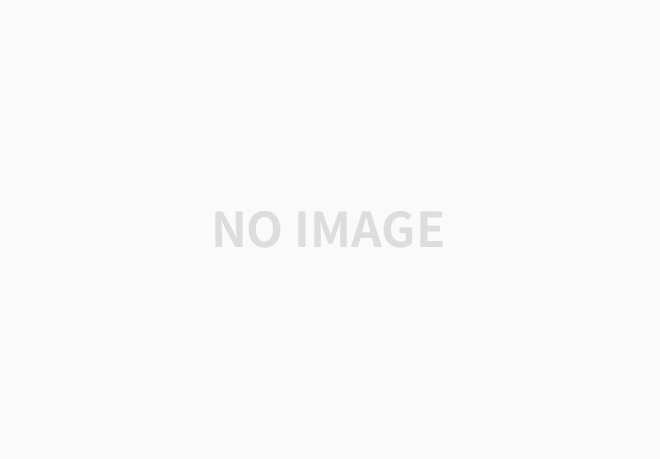
깃헙가서 코드를 보면 이렇게 하드코딩한 String extension입니다.
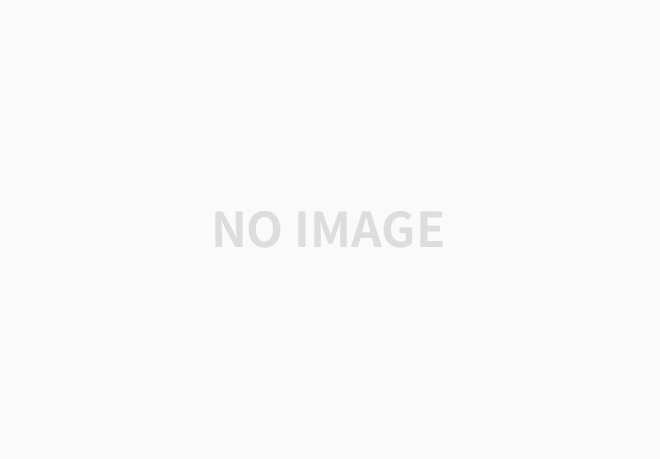
이 패키지를 설치하고 사용해보면 identifier가 아니라 Generation 값이 잘 나오는 것을 볼 수 있습니다.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import 'package:device_info/device_info.dart'; | |
✅ import 'package:ios_utsname_ext/extension.dart'; | |
Future<Map<String, dynamic>> _getDeviceInfo() async { | |
DeviceInfoPlugin deviceInfoPlugin = DeviceInfoPlugin(); | |
Map<String, dynamic> deviceData = <String, dynamic>{}; | |
try { | |
if (Platform.isAndroid) { | |
deviceData = _readAndroidDeviceInfo(await deviceInfoPlugin.androidInfo); | |
} else if (Platform.isIOS) { | |
deviceData = _readIosDeviceInfo(await deviceInfoPlugin.iosInfo); | |
} | |
} catch(error) { | |
deviceData = { | |
"Error": "Failed to get platform version." | |
}; | |
} | |
return deviceData; | |
} | |
Map<String, dynamic> _readAndroidDeviceInfo(AndroidDeviceInfo info) { | |
var release = info.version.release; | |
var sdkInt = info.version.sdkInt; | |
var manufacturer = info.manufacturer; | |
var model = info.model; | |
return { | |
"OS 버전": "Android $release (SDK $sdkInt)", | |
"기기": "$manufacturer $model" | |
}; | |
} | |
Map<String, dynamic> _readIosDeviceInfo(IosDeviceInfo info) { | |
var systemName = info.systemName; | |
var version = info.systemVersion; | |
✅ var machine = info.utsname.machine.iOSProductName; | |
return { | |
"OS 버전": "$systemName $version", | |
"기기": "$machine" | |
}; | |
} | |
// 실행 코드 | |
Map<String, dynamic> deviceInfo = await _getDeviceInfo(); | |
print(deviceInfo) | |
// 실행 결과 | |
// 안드로이드 | |
{ | |
"OS 버전": "Android 11 (SDK 30)" | |
"기기": "Google sdk_gphone_x86_arm" | |
} | |
// iOS | |
{ | |
"OS 버전": "iOS 14.4" | |
✅ "기기": "iPhone11" | |
} | |
[2] App Info
사실 저는 version 정보만 필요해서 pubspec.yaml 파일에서 version 정보를 가져올까 했는데,
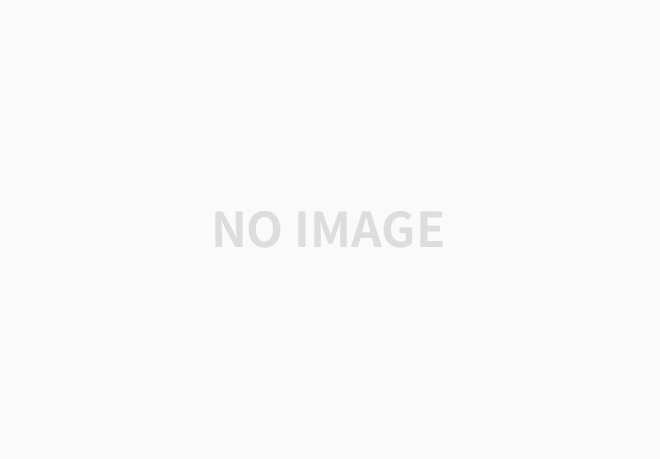
구글링 해보면 다들 package_info 라는 패키지를 쓰시는 것 같아서 이 패키지를 써주었습니다.
이 패키지로 이렇게 4가지 정보를 알 수 있습니다.
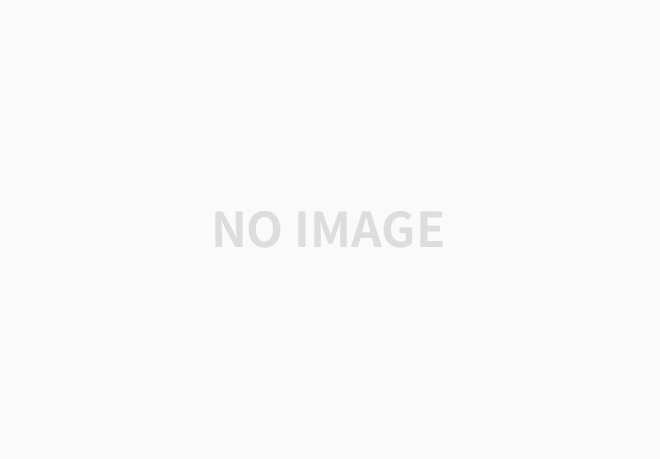
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Future<Map<String, dynamic>> _getAppInfo() async { | |
PackageInfo info = await PackageInfo.fromPlatform(); | |
return { | |
"양파가족 버전": info.version | |
}; | |
} | |
// 실행코드 | |
Map<String, dynamic> appInfo = await _getAppInfo(); | |
print(appInfo) | |
// 실행결과 | |
{ | |
"양파가족 버전": "1.0.2" | |
} |
반응형
'🤼♀️ > Flutter' 카테고리의 다른 글
[Flutter] Marquee Widget 만들기 (2) - scroll (0) | 2021.04.24 |
---|---|
[Flutter] Marquee Widget 만들기 (1) - alternate (1) | 2021.04.23 |
[Flutter] 이메일 보내기 (문의하기) (1) | 2021.03.02 |
[Flutter] Text에 Gradient Color 넣기 (1) | 2020.11.27 |
[Flutter] iOS / 안드로이드 앱 배포하기 (4) | 2020.11.16 |
최근에 올라온 글
최근에 달린 댓글
- Total
- Today
- Yesterday
TAG
- Flutter 로딩
- Flutter Clipboard
- Flutter Text Gradient
- drf custom error
- Dart Factory
- flutter dynamic link
- 장고 Custom Management Command
- Sketch 누끼
- Django FCM
- flutter 앱 출시
- ribs
- Django Firebase Cloud Messaging
- 플러터 얼럿
- flutter build mode
- Python Type Hint
- DRF APIException
- github actions
- ipad multitasking
- flutter deep link
- Django Heroku Scheduler
- PencilKit
- Flutter getter setter
- Flutter Spacer
- Watch App for iOS App vs Watch App
- METAL
- 장고 URL querystring
- SerializerMethodField
- 플러터 싱글톤
- 구글 Geocoding API
- cocoapod
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
글 보관함