Swift Docs > Protocol > Protocol Composition 을 읽다가 새로 알게된 내용! protocol composition 은 one class type 을 포함할 수 있다고 합니다 😲 이는 required superclass를 명시할 때, 사용할 수 있습니다. In addition to its list of protocols, a protocol composition can also contain one class type, which you can use to specify a required superclass. protocol Named { var name: String { get } } class Location { var latitude: Double var longi..
Swift Docs의 Initialization 과 Extensions을 읽으며 알게 된 내용이다. Swift의 Structure은 자동으로 default initializer와 memberwise initializer를 가지게 된다. 다만 custom initializer를 따로 정의하지 않았을 때만!! 예제를 통해 살펴보자. struct Size { var width = 0.0, height = 0.0 } struct Point { var x = 0.0, y = 0.0 } struct Rect { var origin = Point() var size = Size() } let defaultRect = Rect() let memberwiseRect = Rect(origin: Point(x: 2.0, y..
마틴 파울러 > Mocks Aren't Stubs 글 내용이 좋아서 기록합니다. 제가 편한 말(?) 로 바꾼 부분이 많아서 원글을 읽어보시길 추천드려요! # Regular Tests 아래는 일반적인 JUnit 테스트입니다. 스펙은 아래와 같습니다. 주문을 처리할 만큼의 상품이 창고에 있으면 주문이 완료되고 창고의 상품 수량은 해당 수량만큼 감소합니다. 반면 창고에 제품이 충분하지 않으면 주문이 채워지지 않고 창고에 아무 일도 일어나지 않습니다. public class OrderStateTester extends TestCase { private static String TALISKER = "Talisker"; private static String HIGHLAND_PARK = "Highland Park"..
[DI] DI Container, IOC Container 개념과 예제 에서 간단하게 Property Wrapper 를 사용했었는데요, Swift Docs > Properties > Property Wrappers 에서 더 자세한 내용들을 알게 되어서 정리합니다 ✏️ # Property Wrapper 전제조건 Property Wrapper 는 Swift 5.1 에 추가되었고 local stored variable 에만 사용가능합니다 (global variable 또는 computed variable 에서 사용불가) # Property Wrapper 정의하기 Property Wrapper 를 정의하려면 wrappedValue property 를 정의한 structure, enumeration, class ..
Swift Docs > Closures > Autoclosures 를 바탕으로 하고 있습니다. # Autoclosure 란 autoclosure는 함수에 argument로 전달되는 expression 을 감싸기 위해 자동으로 만들어지는 클로저 입니다. (An autoclosure is a closure that’s automatically created to wrap an expression that’s being passed as an argument to a function) 1) autoclosure 를 전달받고 싶으면, 파라미터 타입을 @autoclosure attribute 로 marking 하면 됩니다. 2) autoclosure는 argument 를 가지지 않으며 리턴값이 있어야합니다. 3)..
Swift Docs > Functions 을 읽다가 자주 혼용되는 용어들을 정확하게 잘 구분해서 쓰는 점이 인상깊었다. 나도 잘 리마인드해서 정확한 용어를 쓰도록 해야겠다. # parameter 와 argument parameter (매개변수) 는 함수의 정의에 포함되는 변수. argument (전달인자) 는 함수를 call 할 때 전달하는 실제값. 스택오버플로우의 이 설명이 가장 간단명료한 것 같아서 가져옴 - Parameter is variable in the declaration of function. - Argument is the actual value of this variable that gets passed to function. 아래는 Swift Docs > Functions 의 내용이다..
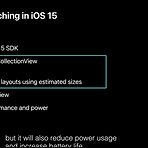
Xcode 13.2 로 iOS 15를 빌드했을 때, 발생하는 문제이다. (iOS 14 이하에서는 발생안함) # 이슈 cell을 토글하면 isSelected가 잘 토글되고 아래 코드도 잘 동작한다. class SomeCollectionViewCell: UICollectionViewCell { override var isSelected: Bool { willSet { if newValue { self.backgroundColor = .red } else { self.backgroundColor = .gray } } } ... } 하지만 사용자의 액션이 아니라 코드로 직접 isSelected 값을 바꾸면 순간적으로는 코드로 세팅해줬던 값으로 바뀌지만, 이전의 isSelected 값으로 다시 원복된다. 디버깅해..
Swift Docs > Concurrency 의 내용을 요약한 글로, 더 자세한 내용은 Meet Swift Concurrency 를 참고하시길 추천드립니다. [ 요약 ] # 개념 ✔️ Asynchronous Function - 일시중단될 수 있으며 그동안 다른 비동기 함수가 해당 스레드에서 실행될 수 있음. ✔️ Asynchronous Sequence - collection의 element를 하나씩 기다릴 수 있음. with for-await-loop ✔️ Asynchronous Function in parallel - 순차적 진행이 아니라 아니라 병렬로 작업을 진행할 수 있음. with async-let ✔️ Tasks and Task Groups - 비동기 코드의 우선순위, 취소 처리에 대해 더 많이..
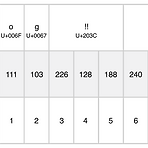
Swift Docs > Strings and Characters 에 나오는 Unicode 관련 내용 입니다. (순서를 조금 재구성하였습니다) # Unicode Swift의 String과 Character는 유니코드를 완벽하게 준수합니다. 또한 세가지 유니코드 표현 (또는 문자열 인코딩) 에 접근할 수 있는 프로퍼티를 제공합니다. 1. UTF-8 Representation - A collection of UTF-8 code units - utf8 property 로 접근 가능. 프로퍼티 타입은 UTF8View (= collection of unsigned 8-bit (UInt8) values) 2. UTF-16 Representation - A collection of UTF-16 code units - ..
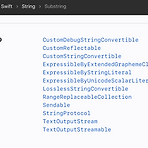
Swift Docs > Strings and Characters > Substring 에 나오는 내용입니다. 애플이 String 연산이 살짝 귀찮아질 것을 감수하고 왜 Substring 타입을 따로 만들었는 지 알 수 있는 내용이여서 좋았습니다! # Substring subscript 또는 prefix method를 이용해서 string의 substring을 구하면 그 타입은 string이 아니라 Substring 입니다. String과 Substring은 둘다 StringProtocol 을 conform 하고 있기 때문에 string에서 쓰던 same methods들을 substring에서도 대부분 사용할 수 있습니다. 즉 substring를 string과 거의 동일한 방식으로 사용할 수 있습니다. S..
- Total
- Today
- Yesterday
- flutter dynamic link
- Flutter Text Gradient
- Python Type Hint
- ribs
- 장고 Custom Management Command
- Flutter 로딩
- flutter deep link
- Flutter Spacer
- Django Heroku Scheduler
- PencilKit
- flutter 앱 출시
- DRF APIException
- Django FCM
- 플러터 싱글톤
- 장고 URL querystring
- Flutter getter setter
- SerializerMethodField
- Sketch 누끼
- Flutter Clipboard
- github actions
- 플러터 얼럿
- drf custom error
- flutter build mode
- Dart Factory
- ipad multitasking
- Django Firebase Cloud Messaging
- cocoapod
- 구글 Geocoding API
- METAL
- Watch App for iOS App vs Watch App
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | |||
5 | 6 | 7 | 8 | 9 | 10 | 11 |
12 | 13 | 14 | 15 | 16 | 17 | 18 |
19 | 20 | 21 | 22 | 23 | 24 | 25 |
26 | 27 | 28 | 29 | 30 | 31 |